Java is the primary language used in Android development. With this, it would be proper to have a good background on the Java development concepts and formats. In this chapter, we will be having a quick overview about Java and its environment.
Java
Java is an object-oriented language, with a syntax similar to the C language. It is structured around objects and methods. A method is an action or something you do with the object.
With the object-oriented concept, this avoids the overly complicated features of C++ that includes operator overloading, pointer, templates, friend class, and many others.
How It Works…
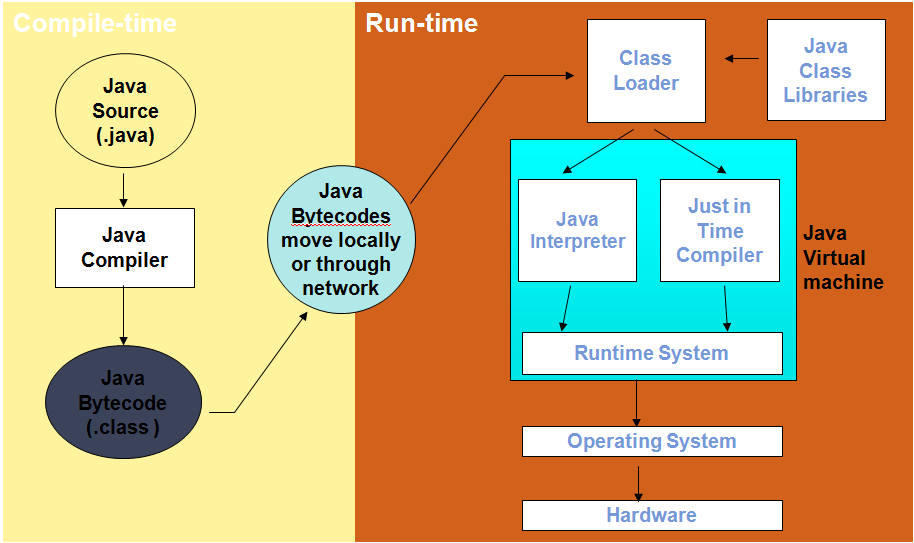
Getting and Using Java
JDK freely downloadable from http://www.oracle.com.
All text editors support java
- Vi/vim, emacs, notepad, wordpad, Sublime, Notepad++, VS Code
- Just save to .java file
Eclipse IDE
- Eclipse
- http://www.eclipse.org
- Android Development Tools (ADT) is a plugin for Eclipse
Android Studio
Compile and Run an Application
- Write java class HelloJava containing a main() method and save in file ”HelloJava.java”
- The file name MUST be the same as class name
- Compile with: javac HelloJava.java
- Creates compiled .class file: HelloJava.class
- Run the program: java HelloJava
- Notice: use the class name directly, no .class!
Hello Java!
File name: HolaWorld.java
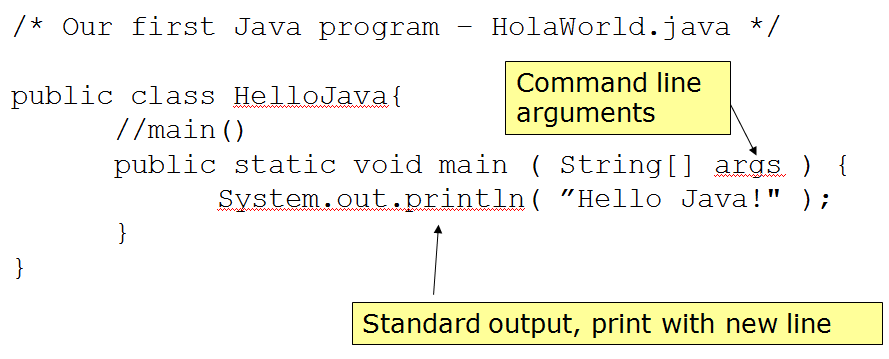
HelloJava in Eclipse
Create a New Project
- File -> New -> Java Project
- Project Name: HelloJava
Add a New Class
- File -> New -> Class
- source folder: HelloJava/src
- Package: excample.test
- Name: HelloJava
- check “public static void main(String[] args)
Write Your Code
- Add your codeSystem.out.println(“Hello Java!”);
Run You Program
- Run -> Run As -> Java Application
Object-Oriented
Java supports OOP
- Polymorphism
- Inheritance
- Encapsulation
Java programs contain nothing but definitions and instantiations of classes
- Everything is encapsulated in a class
Three Principles of OOP
Encapsulation: Objects hide their functions (methods) and data (instance variables)
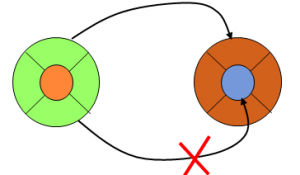
Inheritance: Each subclass inherits all variables of its superclass
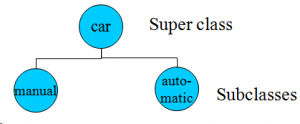
Polymorphism: Interface same despite different data types
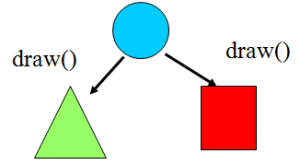
About Class and Object
About Class
- Fundamental unit of Java program
- All java programs are classes
- A class is a template or blueprint for objects
- A class describes a set of objects that have identical characteristics (data elements) and behaviors (methods).Existing classes provided by JREUser defined classes
- Each class defines a set of fields (variables), methods or other classes
What is an Object? An object is an instance of a class. An object has state, behavior and identity.
- Internal variable: store state
- Method: produce behavior
- Unique address in memory: identity
What does it mean to Create an Object? An object is a chunk of memory:
- holds field values
- holds an associated object type
All objects of the same type share code
- they all have same object type, but can have different field values.
Class Definition and Usage
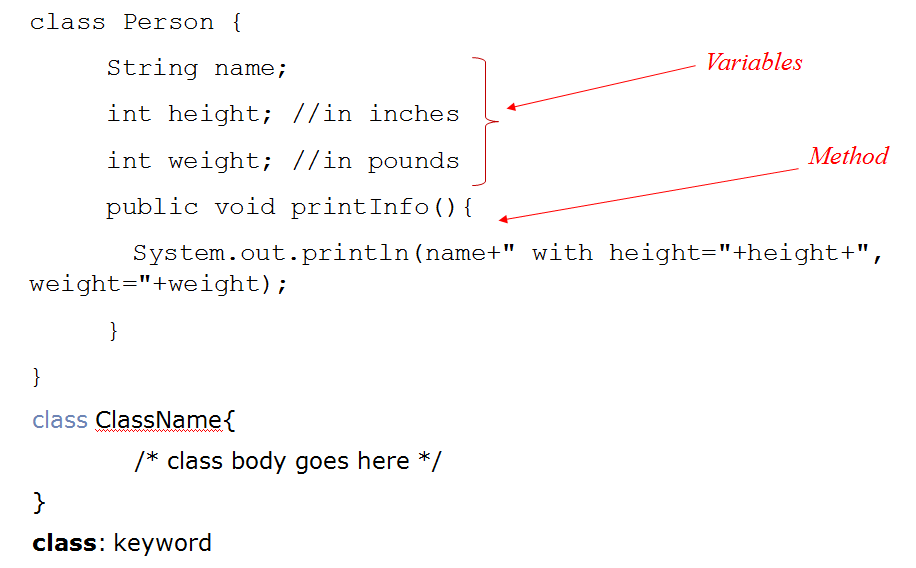
Class Usage
Person john; //declaration
john = new Person();//create an object of Person
john.name= “John Kim”;//access its field
Person sam = new Person();
sam.name=“Sam George”;
john.printInfo(); // call method
sam.printInfo();
Class Reference
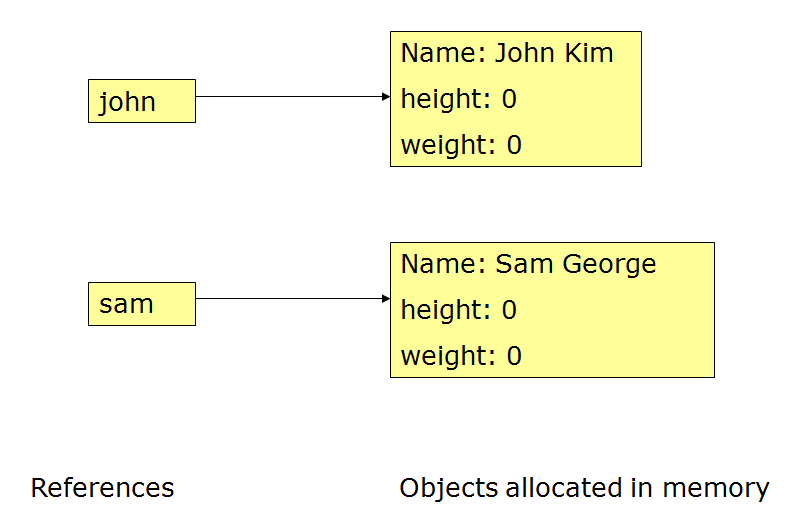
Primitive Data Types
Primitive Types
Primitive type | Size | Minimum | Maximum | Wrapper type |
boolean | 1-bit | — | — | Boolean |
char | 16-bit | Unicode 0 | Unicode 216- 1 | Character |
byte | 8-bit | -128 | +127 | Byte |
short | 16-bit | -215 | +215-1 | Short |
int | 32-bit | -231 | +231-1 | Integer |
long | 64-bit | -263 | +263-1 | Long |
float | 32-bit | IEEE754 | IEEE754 | Float |
double | 64-bit | IEEE754 | IEEE754 | Double |
Reference vs. Primitive
Java handle objects and arrays always by reference.
- classes and arrays are known as reference types.
- Class and array are composite type, don’t have standard size
Java always handle values of the primitive types directly
- Primitive types have standard size, can be stored in a fixed amount of memory
Because of how the primitive types and objects are handles, they behave different in two areas: copy value and compare for equality
Copy
Primitive types get copied directly by =
int x= 10; int y=x;
Objects and arrays just copy the reference, still only one copy of the object existing.
Person john = new Person();
john.name=”John”;
Person x=john;
x.name=”Sam”;
System.out.println(john.name); // print Sam!
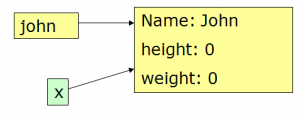
Scope
Scoping in a class
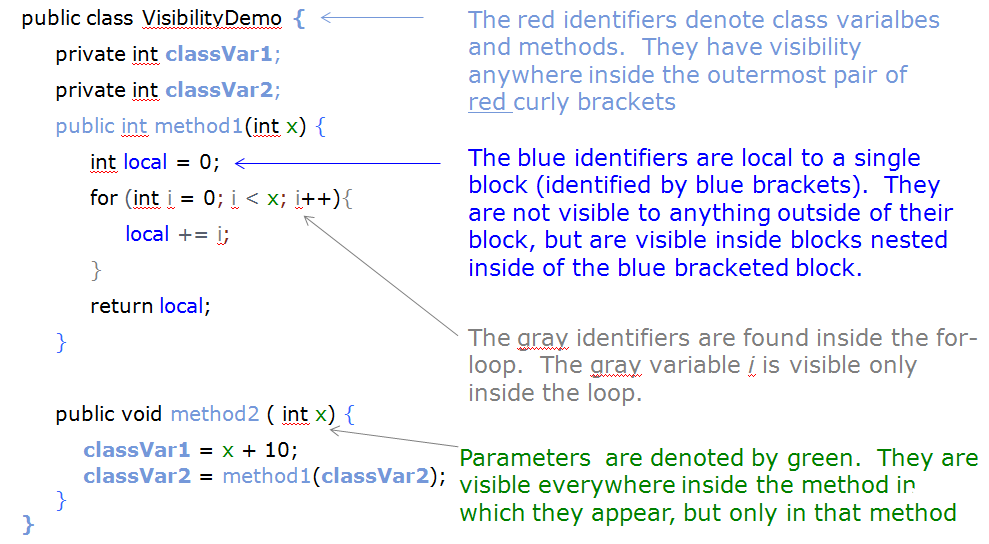
Access Control
Access to packages
- Java offers no control mechanisms for packages.
- If you can find and read the package you can access it
Access to classes
- All top level classes in package P are accessible anywhere in P
- All public top-level classes in P are accessible anywhere
Access to class members (in class C in package P)
- Public: accessible anywhere C is accessible
- Protected: accessible in P and to any of C’s subclasses
- Private: only accessible within class C
- Package: only accessible in P (the default)
Scope Visibility between Classes
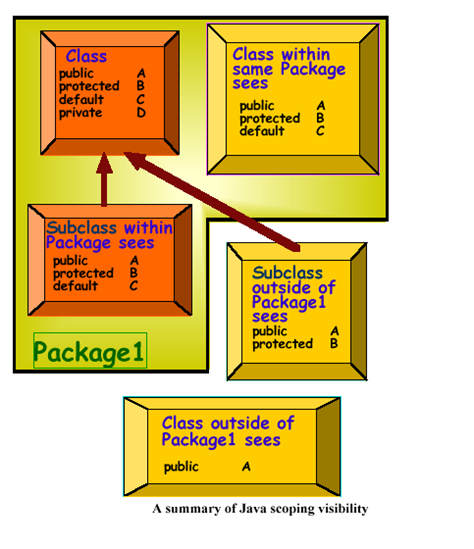
The Static Keyword
Java methods and variables can be declared staticThese exist independent of any objectThis means that a Class’s
- static methods can be called even if no objects of that class have been created and
- static data is “shared” by all instances (i.e., one rvalue per class instead of one per instance
class StaticTest {static int i = 47;}
StaticTest st1 = new StaticTest();
StaticTest st2 = new StaticTest();
// st1.i == st2.I == 47StaticTest.i++;
// or st1.I++ or st2.I++
// st1.i == st2.I == 48