Project Structure
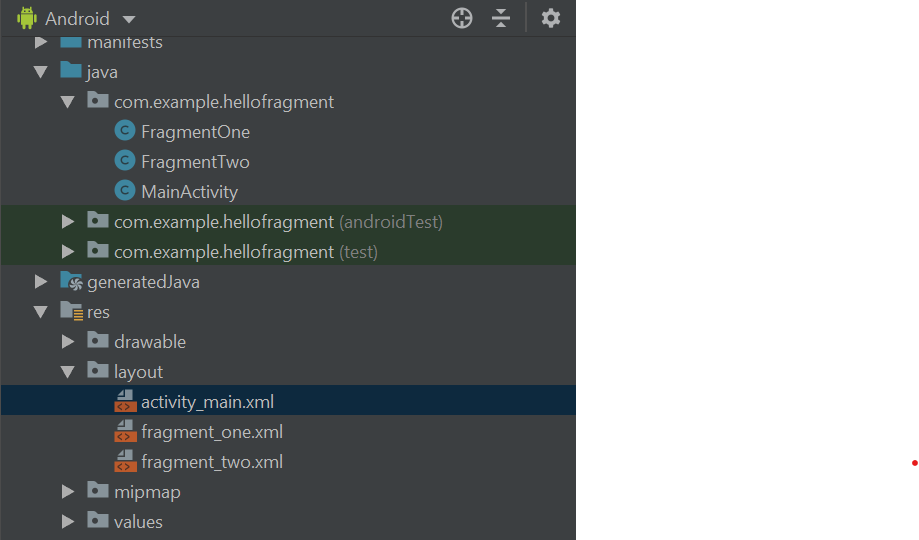
fragment_one.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" android:background="#ffffff"> <TextView android:id="@+id/textView1" android:layout_width="match_parent" android:layout_height="0dp" android:layout_weight="1" android:text="This is fragment One" android:textStyle="bold" /> </LinearLayout>
fragment_two.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" android:background="#00ff00"> <TextView android:id="@+id/textView2" android:layout_width="match_parent" android:layout_height="match_parent" android:text="Fragment Two here!" android:textStyle="bold" /> </LinearLayout>
FragmentOne.java
package com.example.hellofragment; import android.app.Fragment; import android.os.Build; import android.os.Bundle; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; public class FragmentOne extends Fragment { @Override public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) { //Inflate the layout for this fragment return inflater.inflate( R.layout.fragment_one, container, false); } }
FragmentTwo.java
package com.example.hellofragment; import android.app.Fragment; import android.os.Build; import android.os.Bundle; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; public class FragmentTwo extends Fragment{ @Override public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) { // Inflate the layout for this fragment return inflater.inflate( R.layout.fragment_two, container, false); } }
activity_main.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" > <Button android:id="@+id/button1" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="Show Fragment One" android:onClick="selectFrag" /> <Button android:id="@+id/button2" android:layout_width="match_parent" android:layout_height="wrap_content" android:onClick="selectFrag" android:text="Show Fragment Two" /> <fragment android:name="com.example.hellofragment.FragmentOne" android:id="@+id/fragment_place" android:layout_width="match_parent" android:layout_height="match_parent" /> </LinearLayout>
MainActivity.java
package com.example.hellofragment; import android.os.Bundle; import android.view.View; import android.app.Activity; import android.app.Fragment; import android.app.FragmentManager; import android.app.FragmentTransaction; public class MainActivity extends Activity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); } public void selectFrag(View view) { Fragment fr; if(view == findViewById(R.id.button2)) { fr = new FragmentTwo(); }else { fr = new FragmentOne(); } FragmentManager fm = getFragmentManager(); FragmentTransaction fragmentTransaction = fm.beginTransaction(); fragmentTransaction.replace(R.id.fragment_place, fr); fragmentTransaction.commit(); } }
Running Program
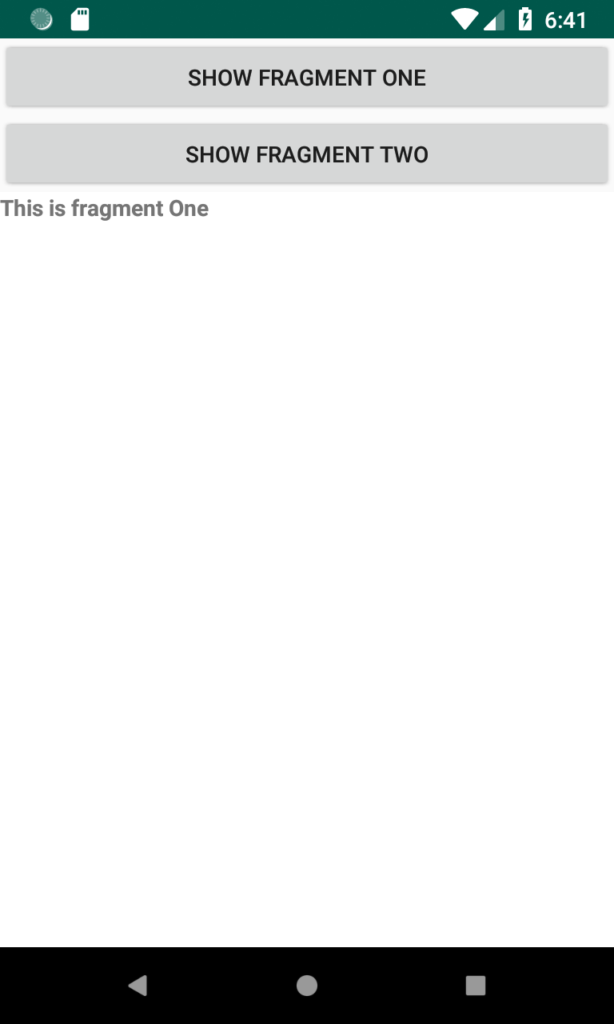
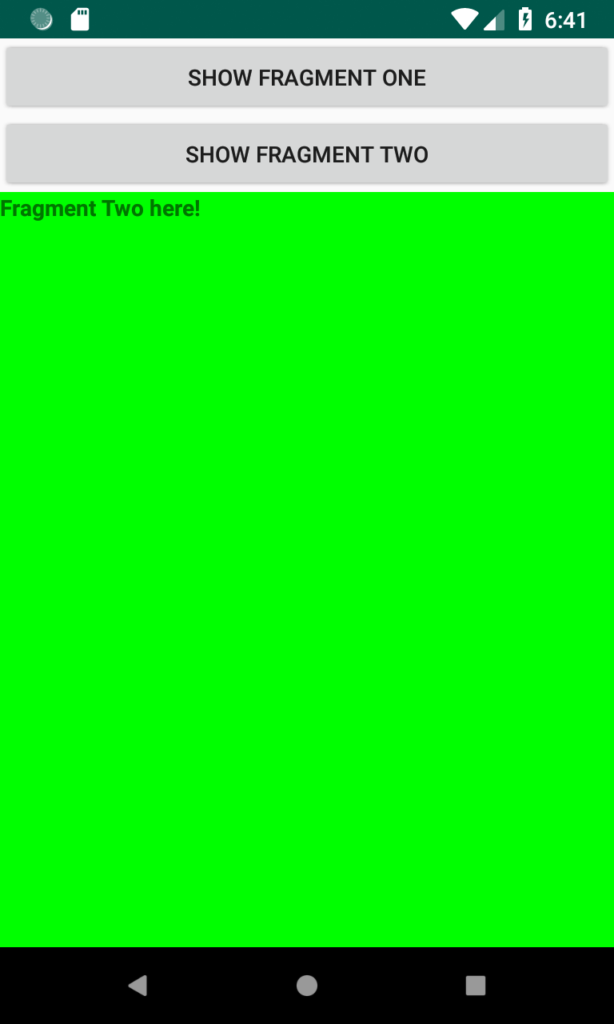